Given a 2D matrix matrix, find the sum of the elements inside the rectangle defined by its upper left corner (row1, col1) and lower right corner (row2, col2).
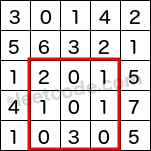
The above rectangle (with the red border) is defined by (row1, col1) = (2, 1) and (row2, col2) = (4, 3), which contains sum = 8.
Example:
Given matrix = [ [3, 0, 1, 4, 2], [5, 6, 3, 2, 1], [1, 2, 0, 1, 5], [4, 1, 0, 1, 7], [1, 0, 3, 0, 5] ] sumRegion(2, 1, 4, 3) -> 8 update(3, 2, 2) sumRegion(2, 1, 4, 3) -> 10
Note:
- The matrix is only modifiable by the update function.
- You may assume the number of calls to update and sumRegion function is distributed evenly.
- You may assume that row1 ≤ row2 and col1 ≤ col2.
Binary indexed tree solution: Time: O(log m * log n), Space: O(m * n)
public class NumMatrix {
//Space: O(m * n)
int[][] tree, arr;
int m;
int n;
public NumMatrix(int[][] matrix) {
m = matrix.length;
if(m==0)return;
n = matrix[0].length;
if(m == 0 || n==0)return;
tree = new int[m+1][n+1];
arr = new int[m][n];
for(int i=0; i<m; ++i){
for(int j=0; j<n; ++j){
update(i, j, matrix[i][j]);
}
}
}
//Time: O(logm * logn)
public void update(int row, int col, int val) {
int dif = val - arr[row][col];
//update new value in element array!
arr[row][col] = val;
//update dif in BIT accumulated sume array!
for(int i = row + 1; i <= m; i += i & (-i)){
for(int j = col + 1; j <=n; j += j & (-j)){
tree[i][j] += dif;
}
}
return;
}
//Time: 4 * O(logm * logn) = O(logm * logn)
public int sumRegion(int row1, int col1, int row2, int col2) {
if(row1<0 || col1<0)return -1;
return getSum(row2, col2) - getSum(row2, col1-1) - getSum(row1-1, col2) + getSum(row1-1, col1-1);
}
public int getSum(int row, int col){
int sum = 0;
for(int i = row + 1; i > 0; i -= i & (-i)){
for(int j = col + 1; j > 0; j -= j & (-j)){
sum += tree[i][j];
}
}
return sum;
}
}
// Your NumMatrix object will be instantiated and called as such:
// NumMatrix numMatrix = new NumMatrix(matrix);
// numMatrix.sumRegion(0, 1, 2, 3);
// numMatrix.update(1, 1, 10);
// numMatrix.sumRegion(1, 2, 3, 4);
No comments:
Post a Comment